“Decisions, decisions, decisions”
Handout: 5_intro_1_conditionals
Booleans and their Operators
A boolean is a data type with only two values: True and False. It’s three main operations are
not # toggles the value of the boolean and # True only if all the operands are True or # True if any of the operants is True
For example: let me try
name = "Peter" age = 12 print("expressions involving name") a = (name == "Peter") print( a ) b = (name == "Mark") print( b ) c = (age == 12) d = (age == 50) print("") print("Not operation") print( b ) # False: it is false that name is Mark print( not b ) # True: the opposite of a print("") print("And operations") print( a ) # True: it is true that name is Peter print( a and c ) # True: it is true that name is Peter and age is 12 print( a and d ) # False: although name is Peter, age is not 50 print("") print("Or operations") print( a or c ) # True: name is Peter so it is true regardless of age print( a or c ) # True: age is 12 so it is true regardless of name print( b or d ) # False: name is not Mark and age is not 50 print("") print("Expression") value = (a and b) or not(a or c or d) print( value )
Now we can take a boolean expression and reduce to its value:
(a and b) or not(a or c or d) = (True and False) or not(True or True or False) = (False) or not(True) = False or False = False
Karel’s Boolean functions
The following are the boolean functions that Karel uses to sense the world:
front_is_clear() # True if there is no wall in front of Karel is_facing_north() # True if Karel is facing North token_here() # True if Karel is on a cell with at least one token has_token() # True if the bag of tokens is not empty at_goal() # True if Karel is at the home square
When we call any of them, it returns a boolean, e.g., looking at the image we have…
front_is_clear() == False # it is False that the front is clear is_facing_north() == True # it is True that Karel is facing north token_here() == True # it is True that Karel is on a token
Conditionals – If / else
Karel uses the sensing functions to make decisions using the ‘if / else’ structure.
The ‘if /else’ structure looks like this:
if: # is True -> run body of the 'if' command 1 # start of the body of the 'if' command 2 ... command n # end of the body of the 'if' else: # is 'False' -> run body of the 'else' command a # start of the body of the 'else' command b ... command z # end of the body of the 'else'
If the condition is True, Karel runs the commands in the body of the if; otherwise, it runs those in the body of the else. The else portion of the structure is optional.
Complementary functions
The sensing functions of Karel are limited and do not allow us to talk to him in a natural way. For example, we usually would not ask “not front_is_clear()” but instead we rather ask “wall_in_front()”. Functions that are the opposite of each other are called complementary functions.
The following are the complementary functions of some of Karel’s built-in sensing functions:
# complement of front_is_clear() def wall_in_front(): return not front_is_clear() # complement of token_here() def cell_is_empty(): return not token_here() # complement of has_token() def bag_is_empty(): return not has_token()
We have added these complementary functions to the library.
Training Missions
TM1 Life in SoCal – Paving the way
Some tiles of the 6-step path to Karel’s house are missing. Karel might have some tiles in his bag but does not know how many he has. Help Karel replace as many tiles as possible, take him home, face him south to see his work, and have him announce he is home.
Answer: Show it to me
A home square that has a small black dot on one of its sides, like in the case of the one on the right, indicates that the robot must finish the mission facing in that direction.
In this mission we use the very useful function safe_put():
def safe_put(): if has_token(): put()
Calling safe_put() instead of put() guarantees that the robot will never try to place a token from an empty bag. We will find this function in our library from now on.
TM2 Life in SoCal – Another touchdown!
Training for the day is almost over. Karel will try to score one more touchdown and then head to the showers. However, his teammates left footballs all over the place.
Karel must run straight toward the end-zone which is not farther than 10 steps. If he finds a ball it is path he must pick it up and score. Of course, if there are more than 1 ball in its path he should still pick only one ball and ignore the others. Have Karel announce whether he found a ball and scored or not.
Answer: Show it to me
The initial setup shows that the number of tokens in each cell will be a number between 0 and 1, i.e., some of the cells will have tokens and some others will not. The value will appear when the ‘Play’ button is pressed.
The 3 robots show the three possible initial positions of the robot. The program will choose one when the ‘Play’ button is pressed.
In this mission we use the very useful functions safe_take() and safe_move():
def safe_take(): if token_here(): take() def safe_move(): if front_is_clear(): move()
that guarantee that the Karel will not try to drive into a wall or try to take a token from an empty cell. We will find these functions in our library from now on.
3rd grade – Strawberries – Dessert
Karel’s 8-cell garden is blossoming with each cell having 0 or 1 strawberries. Have Karel go to his garden and collect as many strawberries as possible, then bring him back home and, finally, orient him to look at his garden.
Answer: Show it to me
In this case, only the 2nd, 3rd and 8th cells ended up 1 strawberry; all the other cells have 0 strawberries.
4th grade – Olympic dreams – Biathlon
In a team everyone helps in any way they can. Today Karel is assisting the biathlon athletes. They arrive to the shooting station tired and with the adrenaline at an all-time high, and have to shoot at 5 targets nested in 5 bowls, knocking them to the floor. Karel’s job is to replace the targets that have been knocked down and to pick up the ones that lie on the floor.
Karel starts the mission with 5 targets in his bag. Of course, we have no idea which bowls will need to be replenished and which targets are going to be knocked down so we must write our code in such a way that it can handle any result. After this, Karel must go back to the home square, ready for the next round of shooters.
Answer: Show it to me
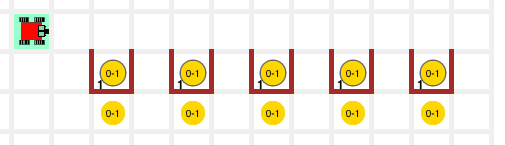
Before…
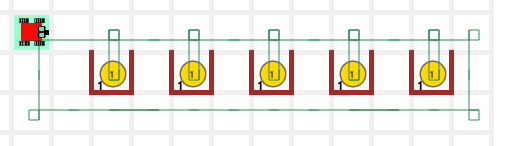
…and after.
Use the safe_take() function from the library.
5th grade – Life in SoCal – Launch Pad
Karel continues with his dream to become an astrodroid. Today he is training his take-off skills. To prepare the rocket for take-off he has to ‘balance the load’, i.e., he has to make sure that there is a single token in each compartment to have the same weight on both sides of the rocket. Then he has to proceed to the cabin and announce he is ready with a “Blast-off!” message. Karel starts the mission with 16 tokens in his bag.
Answer: Show it to me
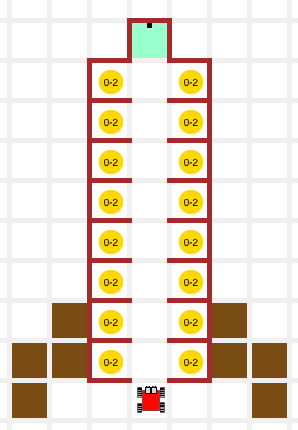
Before..
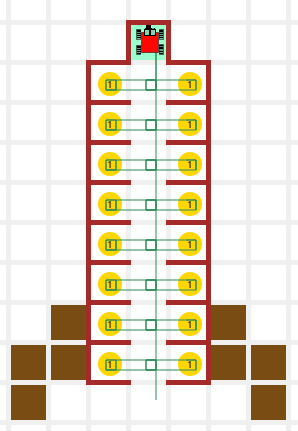
…and after
6th grade – Life in SoCal – Landing Pad
Karel continues with his dream to become an astrodroid. After his successful take-off mission he now has to show that he can land the rocket. He will land it in one of 4 possible pads, at an unknown orientation. Using the known configuration of walls around each path, Karel must figure out where he is with respect to the home square and go to it.
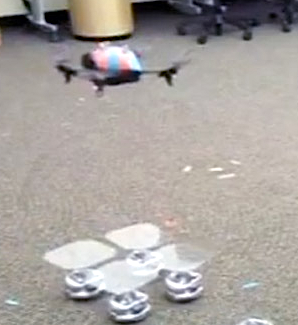
Image from http://www.geeky-gadgets.com/
Answer: Show it to me
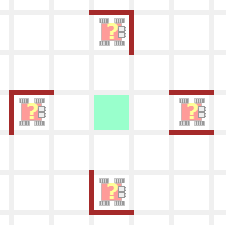
Before..
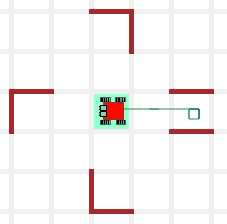
…and after.
Use the sensing function is_facing_north() to orient Karel to the North. Then use the walls to determine the landing pad in which Karel is.