“Computer Science is no more about computers than astronomy is about telescopes”
— Edsger Dijkstra
Handout: 4_intro_1_functions
Custom functions
Custom functions are mini-programs inside our program that we can call ‘later’. We use them to split a problem in independent parts.
Python ignores a function until we ‘call’ it. When we ‘call’ a function, Python looks for its definition and runs its commands in order; when all the instructions have been executed, Python returns to the instructions that called the function.
Python recognizes the commands that are part of the function because they are all indented 4 spaces to the right. We define functions like this:
def foo( ): # define a function name 'foo' command_1 # start of the body of the function command_2 # in Python commands in the body are indented 4 spaces .... command_n # end of the body of the function foo() # calling the function foo()
The function is the single most important concept that you will learn in programming. Some god rules of thumb when writing functions are:
- have your functions do only one thing
- keep your functions short
- give your functions meaningful names
- keep the length of all your functions about the same
Aliases
A function is an alias of another function if it simply renames it. For example, in our library we have the function left() that is an alias of the built-in function turn_left():
def left(): # when left() is called .... turn_left() # it, in turn, calls turn_left()
Since left() is an alias of turn_left(), both do exactly the same. We can use aliases to shorten or clarify function names, and to translate our commands to another language.
Top-down approach to problems
The goal of these two weeks is to learn to solve a problem by splitting it into pieces. At this stage our approach to problem solving is the following:
- Understand what the problem is: e do not rush to write the program before understanding what it is asking us to do.
- Formulate the problem: identify pieces of the problem that are independent of each other.
- Look for patterns: identify the beginning and endings of patterns, if any
- Implement it: write down the sequence of independent parts of the problem. This code should look like english.
- Repeat this process for each of your functions until you can express each solution in terms of functions that already exist, i., either built-ins or library functions.
New functions in the library
The library now has two additional functions:
sidestep_right() # move to the right cell facing in the original direction sidestep_left() # move to the left cell facing in the original direction
Training Missions
TM1 Life in SoCal – Touchdown
In this mission we will use functions to split a problem in independent parts.
Karel needs to pick up the football and score a touchdown. Let’s do it!
Answer: Show it to me
Our main function (the function at the ‘top’ of all others) has to move Karel to the ball, have him take it, then move Karel to the end zone and, finally, have him put the ball down. The following program solves the mission completely:
# main function go_to_ball() pick_ball_up() go_to_endzone() score()
Now that we solved the top function, we work out it of the functions under it: we need to create a function for each of our commands using built-in and custom functions. Two of our functions are aliases:
def pick_ball_up(): take() def score(): put()
The other two of functions are also fairly simple:
def go_to_ball(): repeat(move, 3) def go_to_endzone(): repeat(move, 6)
To get the final program, we put our functions together with the main program. The order in which we place our functions is not important as long as the main function is last:
def go_to_ball(): repeat(move, 3) def go_to_endzone(): repeat(move, 6) def pick_ball_up(): take() def score(): put() # main function go_to_ball() pick_ball_up() go_to_endzone() score()
TM2 Strawberries – Single file
In this mission we will use a function to describe a pattern.
Karel wants to plant 10 strawberry plants in each of the 7 cells in front of his house. Karel starts with 70 plants in his bag. Help Karel be the best farmer he can be and then take him home.
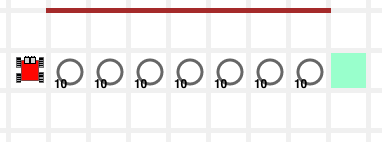
Before: Ready to plant the strawberries
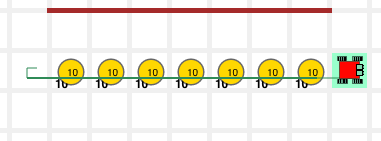
After: All done
Answer: Show it to me
Our main function has to turn Karel to the right, then plant all the strawberries and then go home. The following program solves the ‘top’ problem completely:
# main function right() plant_berries() go_home()
Now let’s work out the functions under our ‘top’ problem. There is no command to turn right but we can effectively turn right if we turn left three times. To make the program more readable, we’ll write an alias to turn_left() and use it to write our right() function:
def left(): turn_left() def right(): repeat( left, 3 )
There are two ways in which we can plant the strawberries. In the first one, we spell out what Karel needs to do; in the second one, we look for the pattern, write a function that describes it, and then use the function. Clearly, the second approach is better than the first:
Version 1:
def pick_up_berries(): move() repeat( put, 10 ) move() repeat( put, 10 ) move() repeat( put, 10 ) move() repeat( put, 10 ) move() repeat( put, 10 ) move() repeat( put, 10 ) move() repeat( put, 10 )
Version 2:
def move_and_put(): move() repeat( put, 10 ) def plant_berries(): repeat( move_and_put, 7 )
After picking up the berries Karel will be in the cell to the left of the home square. Hence, go_home() is simply an alias of move(). The complete final program is:
def left(): turn_left() def right(): repeat( left, 3 ) def move_and_put(): move() repeat( put, 10 ) def plant_berries(): repeat( move_and_put, 7 ) def go_home(): move() # main function right() plant_berries() go_home()
3rd grade – The missing gosling
First the chickens and now the gosling! That pen must have a hole. Have Karel put the gosling back in its pen and then take Karel to his home square.
Answer: Show it to me
Hint: your main program can be:
# main program take_the_token() put_the_token() go_to_home_square() say( "done" )
4th grade – The diagonal garden
Pick up the strawberries and then take Karel home. This program is very short if you use the functions in the library.
Answer: Show it to me
Use functions to split the mission in independent parts. The main function could be something like this:
# main program go_to_berries() repeat(take_berry, 5) go_home()
5th grade – Strawberries – Fertilizer
Now we need fertilizer for the strawberries. Karel just got an order of 8 pallets of 10 bags each, all of which have to be stored in their corresponding sheds. Nasty work but if we want strawberry shortcake we need the strawberries so….
As usual, take advantage of the functions in the library.
Answer: Show it to me
Use a function to describe the pattern.
6th grade – Olympic dreams – Speed skating
You cannot imagine the problem of fitting Karel with skates.. oh well. Karel is heading to the speed skating track: a single clove-shaped lap. Pick up all the 16 flags and then drop them at the podium. Will it be a medal or a contusion?
This is your chance to medal. Good luck.
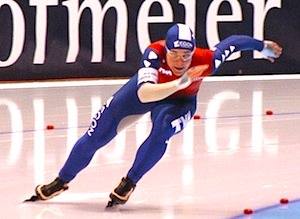
“Paulien van Deutekom (08-12-2007)” by McSmit – Own work. Licensed under Creative Commons Attribution-Share Alike 3.0-2.5-2.0-1.0 via Wikimedia Commons
Answer: Show it to me